Customizing the Gridview
After completion of simple gridview, now I am going to customize that gridview like opening the full-sized images from gridview and naming the images of the gridview.
For this, i create a new android project called "Grid_cus"
As we have done before, drag the gridview layout in graphical layout as shown below:
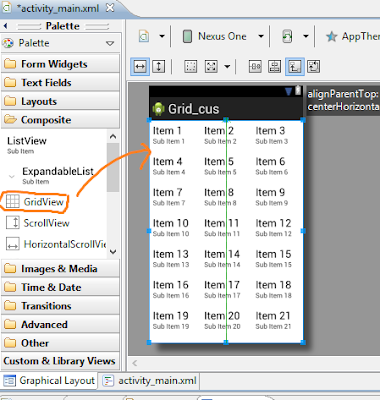 |
Draging Gridview in the layout |
As like before tutorial, place the pictures in res->drawable-hdpi folder.
Then i create a new class called "ImageControl.java" and write these codes :
public class ImageControl extends BaseAdapter{
Context mcontext;
public static int[] ImageIds= { R.drawable.a, R.drawable.a1,R.drawable.a2, R.drawable.a3,
R.drawable.f, R.drawable.e,R.drawable.a7, R.drawable.a6,R.drawable.a5, R.drawable.a4,R.drawable.r, R.drawable.a1,R.drawable.v};
String texts[] = new String[]{"good","best","bad","damn","lool","joker","hard",
"kool","magic","aaare","pop","rock"};
public ImageControl(Context context){
mcontext = context;
}